Email Functions¶
In this section, we will cover the following functions in-depth listed below:
- uadmin.EmailFrom
- uadmin.EmailPassword
- uadmin.EmailSMTPServer
- uadmin.EmailSMTPServerPort
- uadmin.EmailUsername
- uadmin.SendEmail
uadmin.EmailFrom¶
var EmailFrom string
EmailFrom identifies where the email is coming from.
Used in the tutorial:
To assign a value within an application, visit Email From page for an example.
To assign a value in the code, follow this approach:
Go to the main.go and apply the following codes below:
func main(){
// NOTE: This code works only if database does not exist yet.
uadmin.EmailFrom = "myemail@integritynet.biz"
uadmin.EmailUsername = "myemail@integritynet.biz"
uadmin.EmailPassword = "abc123"
uadmin.EmailSMTPServer = "smtp.integritynet.biz"
uadmin.EmailSMTPServerPort = 587
// ----- IF YOU RUN YOUR APPLICATION AGAIN, DO THIS BELOW -----
// Assign Email From in the Settings
setting := uadmin.Setting{}
uadmin.Get(&setting, "code = ?", "uAdmin.EmailFrom")
setting.ParseFormValue([]string{"myemail@integritynet.biz"})
setting.Save()
// Assign Email Username in the Settings
setting = uadmin.Setting{}
uadmin.Get(&setting, "code = ?", "uAdmin.EmailUsername")
setting.ParseFormValue([]string{"myemail@integritynet.biz"})
setting.Save()
// Assign Email Password in the Settings
setting = uadmin.Setting{}
uadmin.Get(&setting, "code = ?", "uAdmin.EmailPassword")
setting.ParseFormValue([]string{"abc123"})
setting.Save()
// Assign Email SMTP Server in the Settings
setting = uadmin.Setting{}
uadmin.Get(&setting, "code = ?", "uAdmin.EmailSMTPServer")
setting.ParseFormValue([]string{"smtp.integritynet.biz"})
setting.Save()
// Assign Email SMTP Server Port in the Settings
setting = uadmin.Setting{}
uadmin.Get(&setting, "code = ?", "uAdmin.EmailSMTPServerPort")
setting.ParseFormValue([]string{"587"})
setting.Save()
// Some codes
}
Let’s go back to the uAdmin dashboard, go to Users model, create your own user account and set the email address based on your assigned EmailFrom in the code above.
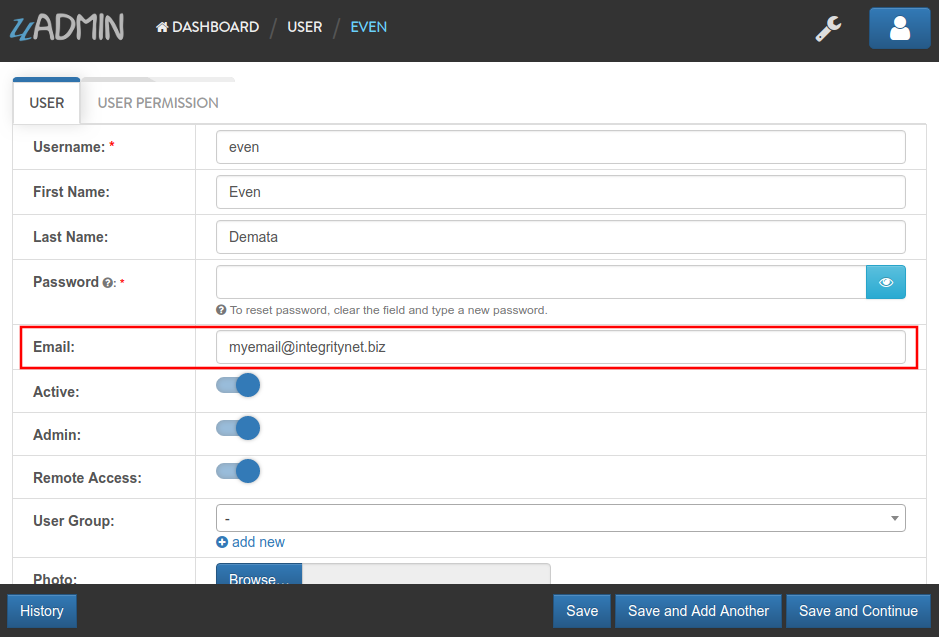
Log out your account. At the moment, you suddenly forgot your password. How can we retrieve our account? Click Forgot Password at the bottom of the login form.
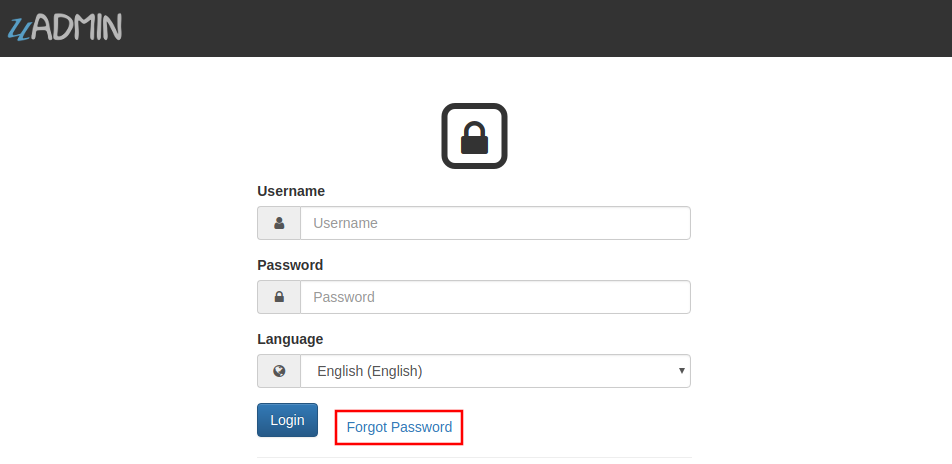
Input your email address based on the user account you wish to retrieve it back.
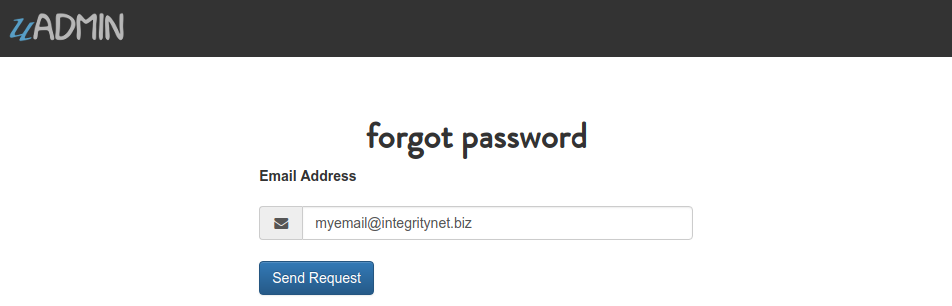
Once you are done, open your email account. You will receive a password reset notification from the Todo List support. To reset your password, click the link highlighted below.
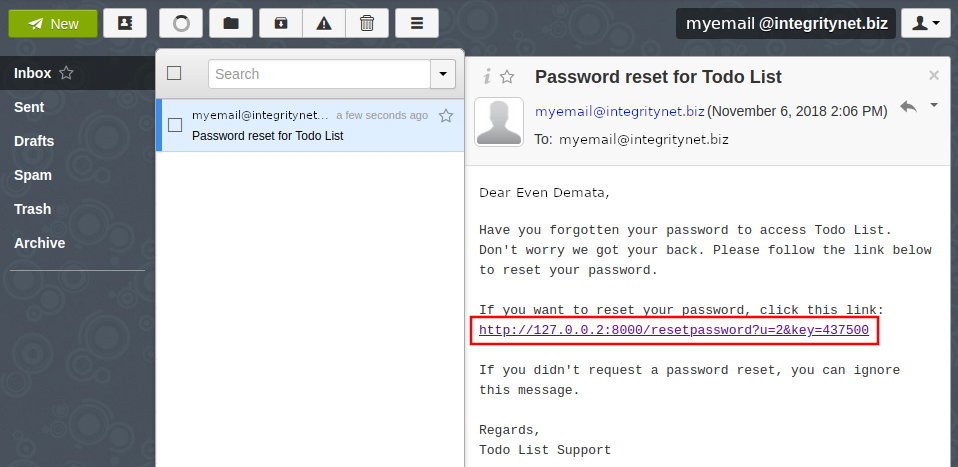
You will be greeted by the reset password form. Input the following information in order to create a new password for you.
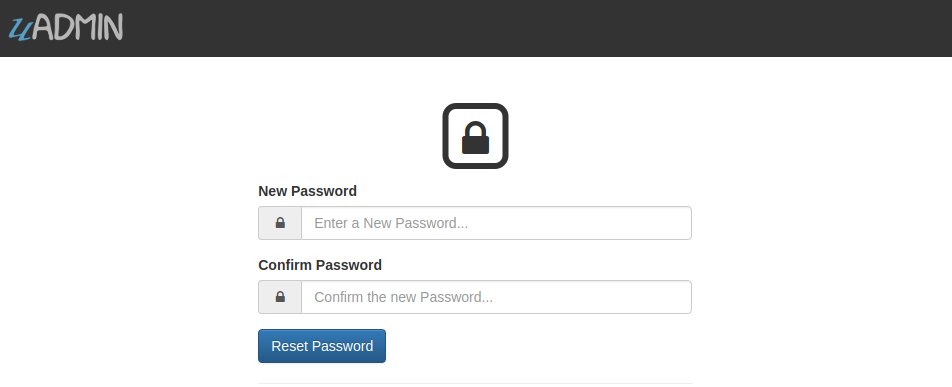
Once you are done, you can now access your account using your new password.
Quiz:
uadmin.EmailPassword¶
var EmailPassword string
EmailPassword sets the password of an email.
To assign a value within an application, visit Email Password page for an example.
To assign a value in the code, visit uadmin.EmailFrom for an example.
uadmin.EmailSMTPServer¶
var EmailSMTPServer string
EmailSMTPServer sets the name of the SMTP Server in an email.
To assign a value within an application, visit Email SMTP Server page for an example.
To assign a value in the code, visit uadmin.EmailFrom for an example.
uadmin.EmailSMTPServerPort¶
var EmailSMTPServerPort int
EmailSMTPServerPort sets the port number of an SMTP Server in an email.
Type:
int
To assign a value within an application, visit Email SMTP Server Port page for an example.
To assign a value in the code, visit uadmin.EmailFrom for an example.
uadmin.EmailUsername¶
var EmailUsername string
EmailUsername sets the username of an email.
See uadmin.EmailFrom for the example.
To assign a value within an application, visit Email Username page for an example.
To assign a value in the code, visit uadmin.EmailFrom for an example.
uadmin.SendEmail¶
func SendEmail(to, cc, bcc []string, subject, body string) (err error)
SendEmail sends email using system configured variables.
Parameters:
to []string: This is who you are primarily writing the email to, it’s clear to both the writer and the recipient who is writing the email and to whom it intended.
cc []string: This means carbon copy and it includes people who might be interested in knowing that there was an email between the sender and the primary TO, typically CC’s are not meant to respond, only the primary sender. Everyone can see who was included in the To and CC.
bcc []string: This means blind carbon copy. The sender has added people that the receiving TO and CC are not able to see as a part of the email, someone on BCC is not to respond and they will not be included in the response from the TO or CC. BCC is often used to include a stakeholder like a boss to make sure they are aware of a situation but they can’t respond. [1]
subject string: This means what your email content is all about.
body string: This means the content of your email. It would be either a job application, the letter of your friend, notifications from your subscribed website, etc.
Go to the main.go and apply the following codes below:
func main(){
// Some codes
// Email configurations
uadmin.EmailFrom = "myemail@integritynet.biz"
uadmin.EmailUsername = "myemail@integritynet.biz"
uadmin.EmailPassword = "abc123"
uadmin.EmailSMTPServer = "smtp.integritynet.biz"
uadmin.EmailSMTPServerPort = 587
// Place it here
uadmin.SendEmail([]string{"myemail@integritynet.biz"}, []string{}, []string{}, "Todo List", "Here are the tasks that I should have done today.")
}
Once you are done, open your email account. You will receive an email from a sender.
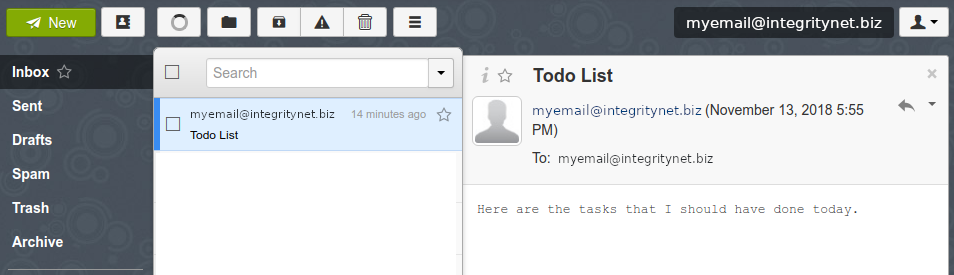
Quiz:
Reference¶
[1] | Corbin, Anke (2017, Feb 27). What is the meaning of TO, CC and BCC in e-mail? Retrieved from https://www.quora.com/What-is-the-meaning-of-TO-CC-and-BCC-in-e-mail |